C Language Interview Questions: C language interview questions with answers are the fundamentals of the influential programming language. These questions will assess a candidate’s knowledge of key concepts such as pointers, memory allocation, control structures, and function handling. An understanding of data types, dynamic memory management, and file handling is crucial. We provide answers. From pointer manipulation to dynamic memory allocation, these questions explore a candidate’s ability to navigate complex programming scenarios. A well-prepared candidate can demonstrate expertise in C language nuances, algorithms, and best practices, ensuring a solid foundation for success in software development roles.
C Language Interview Questions and Answers
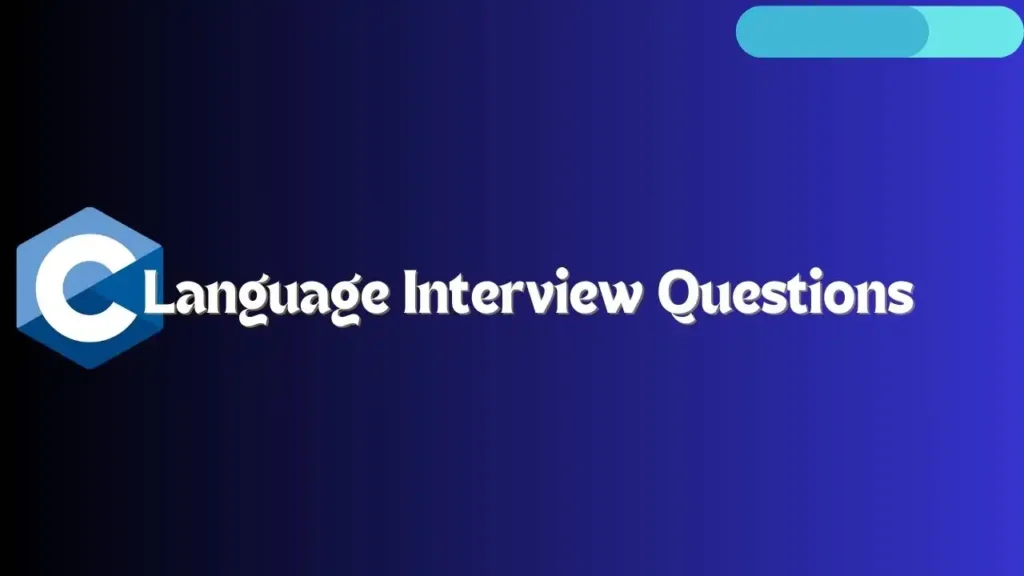
What is C language?
C is a procedural programming language developed by Dennis Ritchie in 1972.
Explain the difference between #include <filename> and #include “filename” in C Language.
#include <filename> searches for the file in the standard system directories, while #include “filename” searches for the file in the current directory first and then in the standard system directories.
What is the purpose of the “main” function in C Language?
The “main” function is the entry point of a C program, and the execution of the program starts from this function.
What is a pointer in C?
A pointer is a variable that stores the memory address of another variable.
Explain the difference between “malloc” and “calloc” in C Language.
Both functions are used for dynamic memory allocation. The key difference is that “malloc” allocates a block of uninitialized memory, while “calloc” allocates a block of memory initialized to zero.
What is the “sizeof” operator used for in C Language?
It is used to determine the size, in bytes, of a variable or data type.
How is a structure different from a union in C Language?
In a structure, each member has its own storage space, while in a union, all members share the same memory space.

What is the purpose of the “typedef” keyword in C Language?
It is used to create an alias (alternative name) for a data type.
Explain the difference between “++i” and “i++”.
Both increment the value of the variable by 1, but “++i” increments the value before the current value is used, and “i++” uses the current value before incrementing.
What is a static variable in C Language?
A static variable retains its value between function calls and has a scope limited to the function in which it is declared.
Read also: The Best 50 MCQ on C Language with Answers
What is a macro in C Language?
A macro is a fragment of code that has been given a name. It is defined using the #define preprocessor directive.
What is the purpose of the “const” keyword in C Language?
It is used to define constants, i.e., variables whose values cannot be modified after initialization.
Explain the difference between “strcpy” and “memcpy”.
“strcpy” is used to copy strings, while “memcpy” is used to copy a specified number of bytes from one memory location to another.
What is the significance of the “&” (address-of) operator in C Language?
It is used to get the address of a variable.
What is a header file in C?
A header file is a file containing declarations of functions, variables, and macros that can be shared across multiple source files.
What is the ternary operator in C?
It is a shorthand way of writing an “if-else” statement. It has the form condition ? expression_if_true : expression_if_false.
What is the purpose of the “volatile” keyword in C Language?
It is used to indicate that a variable may be changed by an external entity such as an interrupt service routine.
Explain the concept of dynamic memory allocation in C Language.
Dynamic memory allocation is the process of allocating memory at run time using functions like “malloc,” “calloc,” and “realloc.”
What is the difference between “break” and “continue” statements in C?
The “break” statement is used to exit from a loop, and the “continue” statement is used to skip the rest of the code inside a loop and move to the next iteration.
What is the purpose of the “enum” keyword in C Language?
It is used to define an enumeration type, which consists of a set of named integer constants.
Explain the concept of a file pointer in C.
A file pointer is a pointer used to navigate through a file. It points to the current position in the file and is used for reading or writing operations.
Read also: 50 Best MCQs On Increment And Decrement Operators In Java
What is the difference between “int” and “unsigned int” in C Language?
“int” can hold both positive and negative values, while “unsigned int” can only hold non-negative values (0 and positive integers).

What is the purpose of the “do-while” loop in C Language?
It is used to create a loop that executes a statement or a block of statements repeatedly until a specified condition becomes false.
Explain the “switch” statement in C.
The “switch” statement is used to select one of many code blocks to be executed, based on the value of an expression.
What is the purpose of the “auto” keyword in C Language?
In modern C, the “auto” keyword is rarely used, but traditionally, it is used to declare automatic variables, which are local variables with a limited scope.
How can you swap the values of two variables without using a temporary variable?
You can use XOR bitwise operation: a = a ^ b; b = a ^ b; a = a ^ b;
What is the purpose of the “return” statement in C Language?
It is used to return a value from a function.
Explain the concept of function prototypes in C Language.
A function prototype is a declaration of a function that tells the compiler about the function’s name, return type, and parameters. It is used to inform the compiler about the existence of a function before it is used.
What is the significance of the “extern” keyword in C?
It is used to declare a global variable or function in another file.
How can you allocate memory for an array dynamically in C Language?
You can use the “malloc” or “calloc” functions to allocate memory for an array dynamically.
What is a bit-field in C?
A bit-field is a data structure used for storing data in a specified number of bits.
Explain the difference between “fopen” and “open” functions in C Language.
“fopen” is a standard C library function used to open a file, while “open” is a system call used for the same purpose in Unix-like operating systems.
What is the purpose of the “const pointer” in C?
It is a pointer that points to a constant value, and once assigned, it cannot be used to modify the value it points to.
What is the purpose of the “static” keyword in C Language?
It has different meanings depending on the context. In the case of a static variable, it makes the variable retain its value between function calls.
Explain the “register” keyword in C.
The “register” keyword suggests to the compiler that a variable will be heavily used and should be stored in a register if possible.
What is the purpose of the “void” data type in C Language?
It is used to indicate that a function does not return any value.
What is the difference between “printf” and “sprintf” in C Language?
“printf” is used to print formatted output to the standard output (usually the console), while “sprintf” is used to store the formatted output as a string in a buffer.
Read also: Best 50 Java Multiple-Choice Questions to Test Your Skills, and Boost Your Knowledge
Explain the concept of recursion in C.
Recursion is a programming technique in which a function calls itself directly or indirectly.
What is the purpose of the “volatile” keyword in C Language?
It informs the compiler that the value of the variable may change at any time without any action being taken by the code that the compiler finds nearby. In C, the volatile
keyword serves as a signal to the compiler that a variable’s value might change unexpectedly, even if the code itself doesn’t modify it directly. This instructs the compiler to avoid certain optimizations that could lead to incorrect behavior or unexpected results.
Here’s why it’s essential:
- Preventing Optimization Issues:
- Compilers often try to optimize code by assuming that variables remain unchanged unless explicitly modified within the code.
- However, in certain scenarios, variables can change due to external factors beyond the code’s control.
- Common Use Cases:
- Hardware Registers: Values in hardware registers can be altered by external hardware or interrupts.
- Shared Memory: Variables in shared memory can be modified by other threads or processes.
- Global Variables in Interrupt Service Routines (ISRs): ISRs can modify global variables, potentially affecting code execution outside the ISR.
How It Works:
- Declaring a Variable as Volatile:
- Use the
volatile
the keyword before the variable’s data type:volatile int sensor_data;
- Use the
- Compiler’s Restrictions:
- The compiler cannot:
- Cache the variable’s value in a register
- Rearrange instructions that access the variable
- Remove seemingly redundant reads or writes
- The compiler cannot:
- Ensuring Correctness:
- This guarantees that the compiler always reads the variable’s value from memory and writes any changes back to memory, ensuring consistency and avoiding unexpected behavior.
Key Points:
volatile
doesn’t guarantee thread safety in multithreaded environments; it only addresses compiler optimizations.- Use
volatile
judiciously, as overuse can hinder optimization and impact performance. - It’s primarily intended for variables that interact with external factors or those shared across multiple execution contexts.
What is the purpose of the “restrict” keyword in C?
The “restrict” keyword is a declaration qualifier that can be used with pointer declarations. It indicates that a pointer is the only reference to the memory it points to.
Explain the concept of a function pointer in C.
A function pointer is a variable that stores the address of a function. It can be used to call a function indirectly through the pointer.
What is the purpose of the “offsetof” macro in C?
It is used to find the offset of a member within a structure or union.
What is a preprocessor directive in C?
A preprocessor directive is a line in your program that starts with a hash symbol (#) and is processed before the actual compilation.
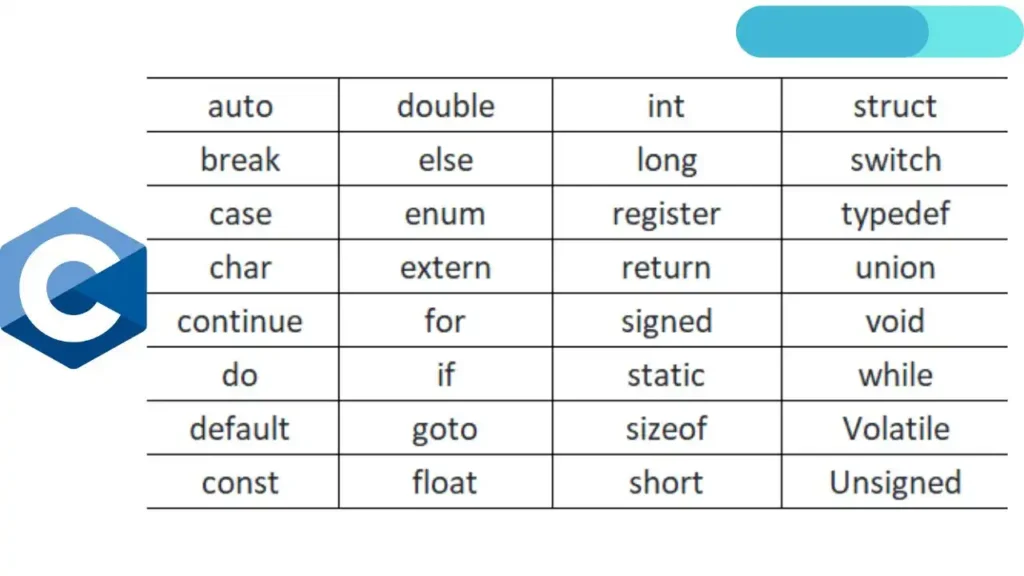
Explain the purpose of the “inline” keyword in C.
The “inline” keyword suggests to the compiler that a function should be expanded in-line at the point of the call. The inline
keyword in C is a suggestion to the compiler to consider expanding a function’s code directly at the point of its call, rather than generating a separate function call. This technique, known as function inlining, can potentially improve performance in certain cases.
Here’s how it works:
- Function Declaration:
- You declare a function as
inline
using theinline
the keyword before its definition:Cinline int add(int x, int y) {
return x + y;
}
- You declare a function as
- Compiler’s Choice:
- The compiler is not obliged to inline the function. It considers factors like:
- Function size (small, simple functions are more likely to be inlined)
- Optimization settings
- Function complexity
- Call Frequency
- The compiler is not obliged to inline the function. It considers factors like:
- Inlining Process:
- If inlined, the compiler copies the function’s code directly into the calling code’s body.
- This eliminates the overhead of:
- Function calls (jumping to the function’s code, passing arguments, returning a value)
Benefits of Inlining:
- Potential Performance Improvement:
- Can reduce function call overhead, especially for frequently called, small functions.
- Improves code locality (code and data closer in memory), potentially enhancing cache performance.
- Code Size Reduction:
- Inlining can sometimes reduce code size by eliminating function call instructions.
- Enables Certain Optimizations:
- Compilers can perform more aggressive optimizations when a function’s entire code is visible at the call site.
Considerations:
- Overuse Can Increase Code Size:
- Inlining large or complex functions can lead to code bloat, potentially hindering performance.
- Might Not Always Improve Performance:
- The compiler’s ability to inline is limited by factors like code complexity and optimization settings.
- Recursive Functions:
- Recursive functions generally cannot be inlined due to the potential for infinite expansion.
Best Practices:
- Use
inline
judiciously:- Prioritize small, frequently called functions that don’t involve recursion or complex logic.
- Trust the compiler:
- Modern compilers often make intelligent inlining decisions without explicit
inline
hints.
- Modern compilers often make intelligent inlining decisions without explicit
- Profile for optimization:
- Use profiling tools to identify performance bottlenecks and selectively apply
inline
where it benefits most.
- Use profiling tools to identify performance bottlenecks and selectively apply
What is the purpose of the “rand” function in C?
It is used to generate pseudo-random numbers in the range 0 to RAND_MAX.
What is the purpose of the “exit” function in C?
It is used to terminate a program and return a value to the operating system.
Explain the concept of a pointer to a function in C.
A pointer to a function is a variable that stores the address of a function. It can be used to call a function indirectly through the pointer.
What is the purpose of the “getchar” and “putchar” functions in C?
“getchar” is used to read a character from the standard input, and “putchar” is used to write a character to the standard output.
Explain the purpose of the “FILE” and “LINE” macros in C.
“FILE” expands to the current filename as a string, and “LINE” expands to the current line number in the source file. In C programming, the __FILE__
and __LINE__
macros provide valuable information about the current source code file and line number, primarily used for debugging and error reporting.
Here’s how they work:
1. __FILE__
Macro:
- Expands to the name of the current source file being compiled, as a string literal.
- It’s typically used to include the file name in error messages or logging statements, making it easier to pinpoint the source of issues.
Example:
#include
int main() {
printf("Running code from file: %s\n", __FILE__);
// Output: Running code from file: main.c
}
2. __LINE__
Macro:
- Expands to the current line number within the source file, as an integer constant.
- It’s often used in conjunction with
__FILE__
to provide precise location information in error messages or logs.
Example:
#include int main() { printf("This code is executing on line number: %d\n", __LINE__); // Output: This code is executing on line number: 4 }
Common Uses:
- Debugging:
- Pinpointing the exact location of bugs or unexpected behavior.
- Providing detailed context in error messages or logs.
- Error Reporting:
- Generating informative error messages that indicate the source file and line where issues occurred.
- Logging:
- Tracking code execution flow and troubleshooting problems.
- Assertions:
- Using
__FILE__
and__LINE__
to create more informative assertion messages.
- Using
- Preprocessor Conditionals:
- Conditional compilation based on source file names or line numbers.
Remember:
- These macros are expanded by the preprocessor, not at runtime.
- They are not variables and cannot be modified directly.
- They’re often used with other debugging tools like debuggers and logging frameworks.
What is the purpose of the “const” keyword in function declarations in C?
It indicates that the function does not modify the values of its parameters.
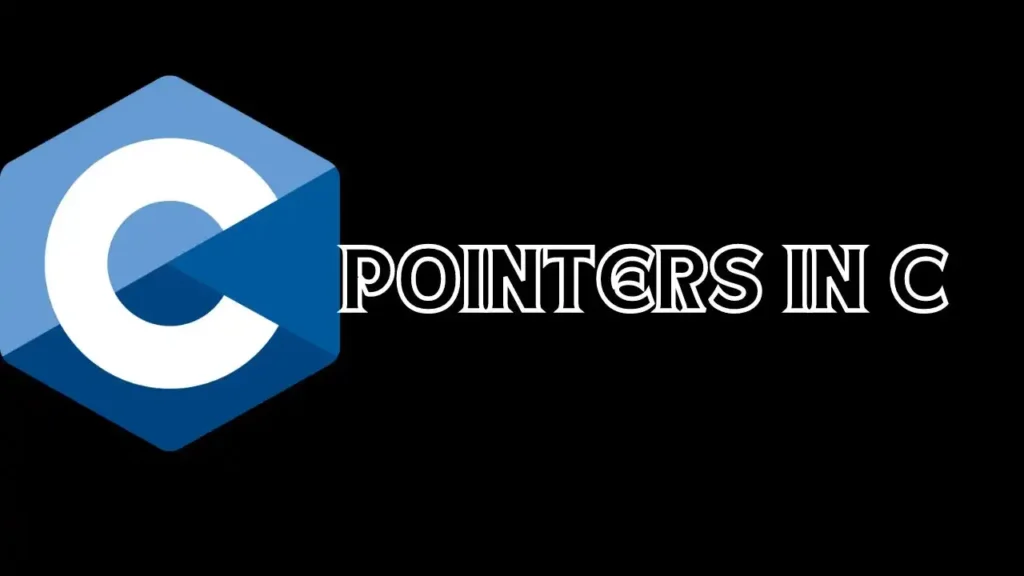
Why is a pointer used in c?
Pointers in C are like digital bookmarks, pointing directly to memory locations where data is stored. They offer a powerful way to manage memory, manipulate data efficiently, and create dynamic structures. Here are key reasons why pointers are essential in C:
1. Direct Memory Access and Manipulation:
- Pointers allow you to access and modify the actual values stored in memory, giving you fine-grained control over data.
- You can create, update, resize, or delete data blocks directly, leading to efficient memory management.
2. Dynamic Memory Allocation:
- Pointers are crucial for allocating memory dynamically at runtime using functions like
malloc()
andcalloc()
. - This means you can create variables and structures as needed, without being limited by predefined memory constraints.
3. Array Manipulation:
- Pointers are closely tied to arrays in C. The array name itself acts as a pointer to the first element’s memory location.
- Using pointers, you can efficiently traverse arrays, access elements, and perform operations like sorting and searching.
4. Function Arguments: Passing by Reference:
- Pointers enable you to pass variables to functions by reference, allowing the function to modify the original variable’s value in the calling code.
- This is more efficient than passing by value, especially for large data structures, as it avoids unnecessary data copying.
5. Returning Multiple Values from Functions:
- While C functions can typically return only one value, pointers can be used to simulate returning multiple values.
- You can pass pointers to variables as arguments, and the function can modify those variables to return multiple results.
6. Data Structures:
- Pointers are indispensable for building complex data structures like linked lists, trees, graphs, and more.
- They enable you to create dynamic relationships between data elements, forming flexible and adaptable structures.
7. System-Level Programming:
- Pointers provide direct access to hardware and memory, making them essential for system-level programming tasks.
- They are used extensively in device driver development, operating system kernels, and low-level programming.