iomanip Library in C++: The iomanip
library in C++ provides a set of functions and manipulators to control the formatting of input and output streams. This library is particularly useful for customizing the way data is presented, making it more readable and aligned according to specific needs. In this post, we’ll explore various manipulators available in the iomanip
library with examples.
To use the iomanip
library, include the <iomanip>
header in your program.
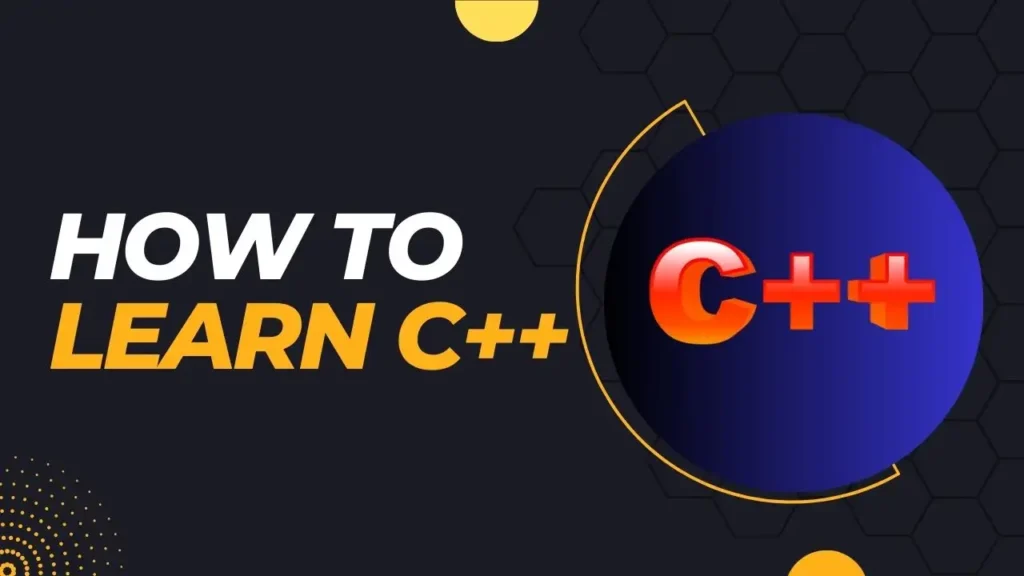
Read also: How To Learn C++: A Beginner’s Guide For 2024, Best Way To Learn C++
Commonly Used iomanip Library in C++
Manipulators
1. setw
The setw manipulator sets the width of the next input/output field.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << “Hello” << std::endl;
std::cout << std::setw(10) << 123 << std::endl;
return 0;
}
Output:
Hello
123
2. setfill
The setfill manipulator sets the character to fill empty spaces when using setw.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setfill(‘*’) << std::setw(10) << “Hello” << std::endl;
std::cout << std::setfill(‘#’) << std::setw(10) << 123 << std::endl;
return 0;
}
Output:
*****Hello
#######123
3. setprecision
The setprecision manipulator sets the decimal precision for floating-point numbers.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::fixed << std::setprecision(2) << 3.14159 << std::endl;
return 0;
}
Output:
3.14
Read also: What are the career opportunities in prompt engineering in 2024?
4. fixed and scientific
The fixed manipulator forces the output of floating-point numbers in fixed-point notation, while scientific forces the scientific
#include <iostream>
#include <iomanip>
int main() {
double num = 1234.56789;
std::cout << std::fixed << num << std::endl;
std::cout << std::scientific << num << std::endl;
return 0;
}
Output:
1234.567890
1.234568e+03
5. showpoint
The showpoint manipulator forces the output to display the decimal point and trailing zeros, even if not necessary.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::showpoint << 123.0 << std::endl;
return 0;
}
Output:
123.000
6. left, right, and internal
These manipulators control the alignment of the output within the field width.
- left: Left-aligns the output.
- right: Right-aligns the output.
- internal: Aligns the output according to the internal formatting.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::left << std::setw(10) << “Hello” << “World” << std::endl;
std::cout << std::right << std::setw(10) << 123 << 456 << std::endl;
return 0;
}
Output:
Hello World
123456
7. boolalpha and noboolalpha
The boolalpha manipulator forces the output of boolean values as true and false instead of 1 and 0, while noboolalpha reverts this behavior.
#include <iostream>
#include <iomanip>
int main() {
bool flag = true;
std::cout << std::boolalpha << flag << std::endl;
std::cout << std::noboolalpha << flag << std::endl;
return 0;
}
Output:
true
1
8. showbase and noshowbase
The showbase manipulator forces the output of numerical values to display the base prefix (e.g., 0x for hexadecimal), while noshowbase reverts this behavior.
#include <iostream>
#include <iomanip>
int main() {
int num = 255;
std::cout << std::showbase << std::hex << num << std::endl;
std::cout << std::noshowbase << num << std::endl;
return 0;
}
Output:
0xff
ff
9. uppercase and nouppercase
The uppercase manipulator forces the output of hexadecimal values to be in uppercase, while nouppercase reverts this behavior.
#include <iostream>
#include <iomanip>
int main() {
int num = 255;
std::cout << std::hex << std::uppercase << num << std::endl;
std::cout << std::nouppercase << num << std::endl;
return 0;
}
Output:
FF
ff
10. hex, dec, and oct
These manipulators set the base for numerical output: hexadecimal, decimal, and octal respectively.
#include <iostream>
#include <iomanip>
int main() {
int num = 255;
std::cout << std::hex << num << std::endl;
std::cout << std::dec << num << std::endl;
std::cout << std::oct << num << std::endl;
return 0;
}
Output:
ff
255
377
11. resetiosflags and setiosflags
The resetiosflags manipulator resets the specified formatting flags, while setiosflags sets the specified formatting flags.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setiosflags(std::ios::showbase | std::ios::hex) << 255 << std::endl;
std::cout << std::resetiosflags(std::ios::showbase | std::ios::hex) << 255 << std::endl;
return 0;
}
Output:
0xff
255
12. internal
The internal manipulator sets the internal alignment for the output. This is useful when you want to align the sign or base prefix to the left while right-aligning the number.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::internal << std::setw(10) << -123 << std::endl;
std::cout << std::internal << std::setw(10) << std::showbase << std::hex << 255 << std::endl;
return 0;
}
Output:
diff
– 123
0x ff
13. showpos and noshowpos
The showpos manipulator forces the output to show the plus sign (+) for positive numbers, while noshowpos reverts this behavior.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::showpos << 123 << std::endl;
std::cout << std::noshowpos << 123 << std::endl;
return 0;
}
Output:
+123
123
14. uppercase and nouppercase
The uppercase manipulator forces the output of letters in scientific and hexadecimal notations to be in uppercase, while nouppercase reverts this behavior.
#include <iostream>
#include <iomanip>
int main() {
double num = 1234.56789;
std::cout << std::uppercase << std::scientific << num << std::endl;
std::cout << std::nouppercase << std::hex << 255 << std::endl;
return 0;
}
Output:
1.234568E+03
ff
15. showpoint
The showpoint manipulator forces the output to display the decimal point and trailing zeros for floating-point numbers.
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::showpoint << 123.0 << std::endl;
std::cout << std::noshowpoint << 123.0 << std::endl;
return 0;
}
Output:
123.000
123
16. fixed and scientific
The fixed manipulator forces the output of floating-point numbers in fixed-point notation, while scientific forces the scientific notation.
#include <iostream>
#include <iomanip>
int main() {
double num = 1234.56789;
std::cout << std::fixed << num << std::endl;
std::cout << std::scientific << num << std::endl;
return 0;
}
Output:
1234.567890
1.234568e+03
17. hexfloat and defaultfloat
The hexfloat manipulator forces the output of floating-point numbers in hexadecimal format, while defaultfloat reverts the format to the default floating-point notation.
#include <iostream>
#include <iomanip>
int main() {
double num = 1234.56789;
std::cout << std::hexfloat << num << std::endl;
std::cout << std::defaultfloat << num << std::endl;
return 0;
}
Output:
0x1.34a46ep+10
1234.56789
18. quoted
The quoted manipulator allows you to handle strings with embedded spaces and quotes more easily. It surrounds the string with double quotes and escapes embedded quotes.
#include <iostream>
#include <iomanip>
int main() {
std::string text = “He said, \”Hello, World!\””;
std::cout << std::quoted(text) << std::endl;
return 0;
}
Output:
“He said, \”Hello, World!\””
In A table iomanip Library in C++
Manipulator | Description | Example Code | Output |
---|---|---|---|
setw | Sets the width of the next input/output field | std::setw(10) << "Hello" | Hello |
setfill | Sets the character to fill empty spaces when using setw | std::setfill('*') << std::setw(10) << "Hello" | *****Hello |
setprecision | Sets the decimal precision for floating-point numbers | std::fixed << std::setprecision(2) << 3.14159 | 3.14 |
fixed | Forces the output of floating-point numbers in fixed-point notation | std::fixed << num | 1234.567890 |
scientific | Forces the output of floating-point numbers in scientific notation | std::scientific << num | 1.234568e+03 |
showpoint | Forces the output to display the decimal point and trailing zeros | std::showpoint << 123.0 | 123.000 |
left | Left-aligns the output within the field width | std::left << std::setw(10) << "Hello" | Hello |
right | Right-aligns the output within the field width | std::right << std::setw(10) << 123 | 123 |
internal | Aligns the output according to the internal formatting | std::internal << std::setw(10) << -123 | - 123 |
boolalpha | Forces the output of boolean values as true and false instead of 1 and 0 | std::boolalpha << true | true |
noboolalpha | Reverts the output of boolean values to 1 and 0 instead of true and false | std::noboolalpha << true | 1 |
showbase | Forces the output of numerical values to display the base prefix | std::showbase << std::hex << 255 | 0xff |
noshowbase | Reverts the output to not display the base prefix | std::noshowbase << std::hex << 255 | ff |
uppercase | Forces the output of hexadecimal values to be in uppercase | std::hex << std::uppercase << 255 | FF |
nouppercase | Reverts the output of hexadecimal values to be in lowercase | std::hex << std::nouppercase << 255 | ff |
hex | Sets the base for numerical output to hexadecimal | std::hex << 255 | ff |
dec | Sets the base for numerical output to decimal | std::dec << 255 | 255 |
oct | Sets the base for numerical output to octal | std::oct << 255 | 377 |
resetiosflags | Resets the specified formatting flags | `std::resetiosflags(std::ios::showbase | std::ios::hex)` |
setiosflags | Sets the specified formatting flags | `std::setiosflags(std::ios::showbase | std::ios::hex) << 255` |
showpos | Forces the output to show the plus sign (+ ) for positive numbers | std::showpos << 123 | +123 |
noshowpos | Reverts the output to not show the plus sign (+ ) for positive numbers | std::noshowpos << 123 | 123 |
hexfloat | Forces the output of floating-point numbers in hexadecimal format | std::hexfloat << 1234.56789 | 0x1.34a46ep+10 |
defaultfloat | Reverts the output of floating-point numbers to the default floating-point notation | std::defaultfloat << 1234.56789 | 1234.56789 |
quoted | Surrounds the string with double quotes and escapes embedded quotes | std::quoted("He said, \"Hello, World!\"") | "He said, \"Hello, World!\"" |
These additional manipulators from the iomanip library provide even more control over the formatting of your C++ input and output, allowing you to create more sophisticated and professional-looking programs.