Mastering Java Basics: Java is one of the most popular programming languages in the world. Developers love it for its simplicity, robustness, and versatility. You can build a desktop application, mobile app, or enterprise-level software. Java provides the tools and features to create powerful and scalable solutions. This article is a beginner-friendly guide to core Java concepts, including an introduction to Java, variables, control structures, methods, and arrays.

Mastering Java Basics Introduction to Java
Java is a high-level, object-oriented programming language designed to be simple, secure, and portable. It is platform-independent, meaning a Java program can run on any machine with a Java Virtual Machine (JVM). Its key features such as robustness, multithreading, and memory management make Java a go-to choice for developers worldwide. Learn how Java programs are compiled and executed, and explore the role of tools like JDK, JRE, and JVM.
Variables in Java
Variables are the building blocks of any programming language. In Java, variables allow you to store and manipulate data efficiently. This section dives into the different types of variables (local, instance, and static), the nuances of data types like int, float, and double, and how to declare, initialize, and use constants. Additionally, discover the concept of variable scope and how it impacts program behavior.
Decision-Making in Java: If-Else and Switch Case
Control structures like if-else and switch are essential for making decisions in Java programs. This section explores:
- The difference between if-else statements and if-else if ladders.
- Logical operators (&&, ||) and their role in decision-making.
- Practical examples, include checking whether a number is even or odd and displaying month names based on numeric input.
Methods in Java
Methods are reusable blocks of code that enhance modularity and readability in Java programs. Learn about:
- The difference between static and non-static methods.
- Method overloading and its applications.
- Passing arguments by value and understanding return types.
- Practical examples such as finding the GCD, checking for prime numbers, and reversing a string. Explore how methods make programming more organized and efficient.
Arrays in Java
Arrays in Java are powerful data structures in Java, allowing you to store and manipulate collections of data efficiently. This section covers:
- How to declare, initialize, and access elements in arrays.
- Single-dimensional vs. multi-dimensional arrays.
- Advanced concepts like jagged arrays and the Arrays utility class. Practical examples include adding matrices, finding the largest and smallest numbers, sorting arrays, removing duplicates, and rotating arrays.
Java Questions and Answers
1. What is the Java Virtual Machine (JVM) and its role in Java?
Java Virtual Machine (JVM) is an abstract machine that provides a runtime environment to execute Java programs. It is responsible for:
- Loading, verifying, and executing bytecode (compiled Java code).
- Managing memory (via Garbage Collection).
- Providing a secure runtime environment for Java applications.
- Translating bytecode into machine-specific instructions.
Read also: 50 Best MCQs on Increment and Decrement Operators in Java
2. Explain the difference between JDK, JRE, and JVM(Java Virtual Machine).
- JDK (Java Development Kit):
- A complete software development kit includes tools for compiling, debugging, and running Java programs.
- Contains JRE and development tools like javac, javadoc, and javap.
- JRE (Java Runtime Environment):
- Provides libraries and JVM required to run Java applications.
- It does not include development tools.
- JVM (Java Virtual Machine):
- Part of JRE, responsible for executing the bytecode.
- It ensures platform independence.
3. What are the key features of Java?
- Platform-Independent: Write once, run anywhere (WORA).
- Object-Oriented: Everything in Java is treated as an object.
- Simple: Easy to learn with simple syntax.
- Secure: Provides runtime security features like bytecode verification.
- Robust: Has strong memory management and exception-handling mechanisms.
- Multithreaded: Supports concurrent execution of programs.
- High Performance: Uses Just-In-Time (JIT) compiler for efficient execution.
- Distributed: Facilitates distributed computing with tools like RMI and EJB.
4. Why is Java called platform-independent?
Java is platform-independent because the source code is compiled into bytecode, which can be executed on any machine with a JVM, regardless of the underlying hardware or operating system. This eliminates the dependency on specific platforms for running Java programs.
5. Explain the process of compiling and running a Java program.
- Write the Java Code:
- Create a .java file using a text editor or IDE.
- Compile the Code:
- Use javac <filename>.java to compile the code. This generates a .class file (bytecode).
- Run the Program:
- Execute the bytecode using java <classname>.
- JVM interprets the bytecode and runs the program.
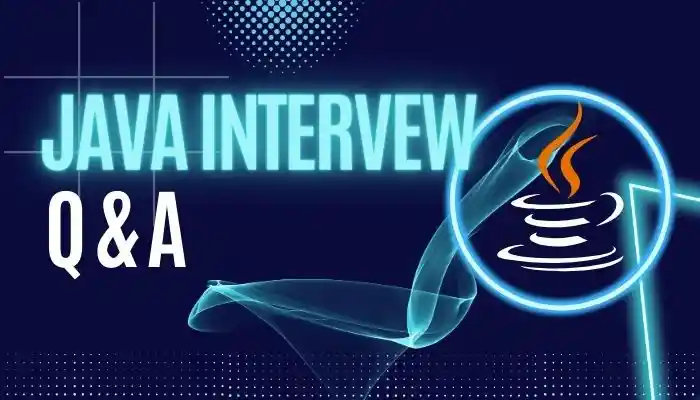
6. What is the main method in Java, and why is it necessary?
The main method is the entry point of a Java program. Its syntax is:
public static void main(String[] args) {
// Code to execute
}
- public: Makes the method accessible to the JVM(Java Virtual Machine).
- static: Allows JVM to invoke the method without creating an object.
- void: Indicates the method does not return a value.
- String[] args: Used to accept command-line arguments.
Without the main method, the JVM would not know where to start executing the program.
7. Write a simple “Hello, World!” program in Java.
public class HelloWorld {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
}
Explanation:
- public class HelloWorld: Declares a class named HelloWorld.
- public static void main(String[] args): The main method where the program starts.
- System.out.println(“Hello, World!”);: Prints “Hello, World!” to the console.
8. What are the different types of variables in Java? Explain each with examples.
In Java, variables are classified into three types:
- Instance Variables:
- Declared inside a class but outside any method, constructor, or block.
- Belong to an instance of the class, and each object has its own copy.
- Default values are assigned if not initialized explicitly.
Example:
class Person {
String name; // Instance variable
int age; // Instance variable
}
- Static Variables:
- Declared with the static keyword inside a class.
- Shared among all objects of the class.
- Have a single copy that is shared across instances.
Example: program in Java
class Counter {
static int count = 0; // Static variable
}
- Local Variables:
- Declared inside a method, constructor, or block.
- Only accessible within that block or method.
- Must be initialized before use.
Example:
class Example {
void display() {
int localVariable = 10; // Local variable
System.out.println(localVariable);
}
}
9. What is the difference between int, float, and double data types in Java?
Data Type | Size | Precision | Use Case |
int | 4 bytes (32 bits) | No fractional values | Whole numbers, e.g., 10. |
float | 4 bytes (32 bits) | Up to 7 decimal places | Decimal values, e.g., 3.14f. |
double | 8 bytes (64 bits) | Up to 15 decimal places | High-precision decimals, e.g., 3.1415926535. |
Example:
int age = 25;
float price = 19.99f;
double pi = 3.1415926535;
10. How does variable declaration differ from initialization in Java?
- Declaration:
- Declaring a variable means specifying its type and name without assigning it a value.
- Example: int number;
- Initialization:
- Assigning an initial value to a declared variable.
- Example: number = 10;
- Combined Declaration and Initialization:
- Declaring and assigning a value in the same statement.
- Example: int number = 10;
11. What are constants in Java? How can you define them?
Constants are variables whose value cannot be changed once assigned. They are declared using the final keyword.
Example:
final double PI = 3.14159; // PI is a constant
PI = 3.14; // This will cause an error
Characteristics:
- Declared with final.
- Typically, constants are written in uppercase by convention.
12. What is the scope of a variable in Java? Explain with an example.
The scope of a variable determines where it can be accessed or modified in the program.
- Local Scope:
Variables declared inside a method or block are only accessible within that method or block.
Example:program in Java
void display() {
int x = 10; // Local variable
System.out.println(x);
}
- Instance Scope:
Instance variables are accessible within the entire class but require an object to access them outside the class.
Example:
class Person {
String name; // Instance variable
void display() {
System.out.println(name);
}
}
- Class/Static Scope:
Static variables are accessible throughout the class and can be accessed using the class name.
Example:
class Counter {
static int count = 0; // Static variable
void increment() {
count++;
}
}
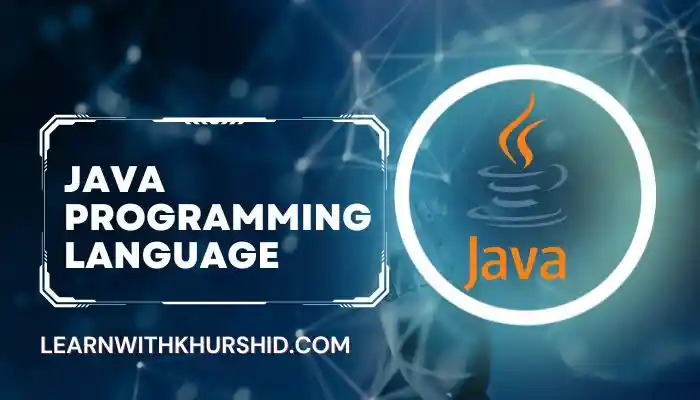
13. Write a Java program to check if a number is positive, negative, or zero using if-else if.
Program in Java
import java.util.Scanner;
public class NumberCheck {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter a number: “);
int number = scanner.nextInt();
if (number > 0) {
System.out.println(“The number is positive.”);
} else if (number < 0) {
System.out.println(“The number is negative.”);
} else {
System.out.println(“The number is zero.”);
}
}
}
Read also: Best 50 Java Multiple-Choice Questions to Test Your Skills, and Boost Your Knowledge
14. How does the if-else statement differ from the if-else if ladder in Java?
Aspect | if-else Statement | if-else if Ladder |
Purpose | Handles a binary condition: one if and one else. | Handles multiple conditions in a sequential manner. |
Structure | Only one if and one else block. | Uses multiple else if blocks between if and else. |
Example | Checks if a number is positive or not. | Checks if a number is positive, negative, or zero. |
Readability | Simpler for binary decisions. | Better for multi-condition checks. |
Examples:
- if-else:
if (x > 0) {
System.out.println(“Positive”);
} else {
System.out.println(“Not positive”);
}
- if-else if ladder:
if (x > 0) {
System.out.println(“Positive”);
} else if (x < 0) {
System.out.println(“Negative”);
} else {
System.out.println(“Zero”);
}
15. Explain the use of logical operators (&&, ||) in Java with an if-else example.
Logical Operators in Java:
- && (Logical AND):
- Returns true if both conditions are true.
- Example: (a > 0 && b > 0) is true only if a > 0 and b > 0.
- || (Logical OR):
- Returns true if at least one condition is true.
- Example: (a > 0 || b > 0) is true if either a > 0 or b > 0.
Example program in Java:
public class LogicalOperatorsExample {
public static void main(String[] args) {
int age = 25;
int salary = 45000;
if (age > 18 && salary > 40000) {
System.out.println(“Eligible for loan.”);
} else if (age > 18 || salary > 40000) {
System.out.println(“Partially eligible for loan.”);
} else {
System.out.println(“Not eligible for loan.”);
}
}
}
16. Write a Java program to check whether a number is even or odd using if-else.
Program in Java
import java.util.Scanner;
public class EvenOddCheck {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter a number: “);
int number = scanner.nextInt();
if (number % 2 == 0) {
System.out.println(“The number is even.”);
} else {
System.out.println(“The number is odd.”);
}
}
}
17. Explain the syntax and use of the switch statement in Java.
The switch statement in Java is used to execute one of many blocks of code based on the value of an expression. It is often used when you have multiple potential values to check against, rather than using multiple if-else statements.
Syntax: program in Java
switch (expression) {
case value1:
// Code block for value1
break; // Exits the switch statement
case value2:
// Code block for value2
break; // Exits the switch statement
// More cases can be added
default:
// Code block if no match is found
}
- expression: The variable or expression whose value is being checked.
- case value: The possible values that the expression can take.
- break: Exits the switch block once a case is matched.
- default: Optional. The block executed if no case matches the value.
18. Write a program in Java to display the name of the month based on a number (1 for January, 2 for February, etc.) using switch.
Program in Java
import java.util.Scanner;
public class MonthName {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter the month number (1-12): “);
int month = scanner.nextInt();
switch (month) {
case 1:
System.out.println(“January”);
break;
case 2:
System.out.println(“February”);
break;
case 3:
System.out.println(“March”);
break;
case 4:
System.out.println(“April”);
break;
case 5:
System.out.println(“May”);
break;
case 6:
System.out.println(“June”);
break;
case 7:
System.out.println(“July”);
break;
case 8:
System.out.println(“August”);
break;
case 9:
System.out.println(“September”);
break;
case 10:
System.out.println(“October”);
break;
case 11:
System.out.println(“November”);
break;
case 12:
System.out.println(“December”);
break;
default:
System.out.println(“Invalid month number.”);
}
}
}
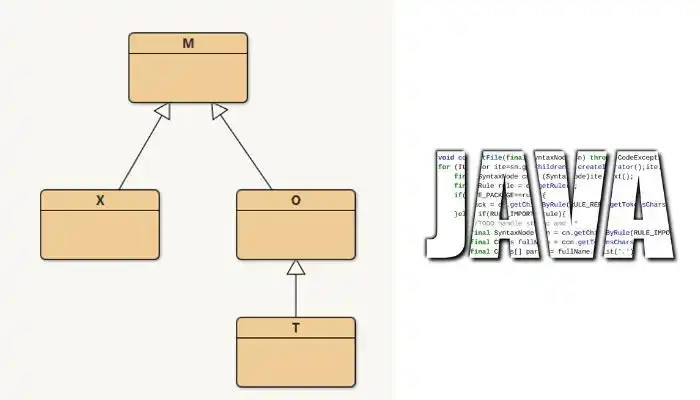
19. Can a switch in Java handle String values? Write an example program.
Yes, starting from Java 7, the switch statement can handle String values as well. The expression in the switch statement can be a string, and the cases can match specific string values.
Example program:
import java.util.Scanner;
public class SwitchStringExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter a day of the week (e.g., Monday): “);
String day = scanner.nextLine();
switch (day.toLowerCase()) {
case “monday”:
System.out.println(“Start of the workweek.”);
break;
case “wednesday”:
System.out.println(“Midweek.”);
break;
case “friday”:
System.out.println(“End of the workweek.”);
break;
case “saturday”:
case “sunday”:
System.out.println(“Weekend.”);
break;
default:
System.out.println(“Invalid day.”);
}
}
}
Read also: Difference between HashSet vs TreeSet in Java, Best Tutorial of HashSet vs TreeSet 2024
20. What happens if a switch statement does not have a break keyword in one of the cases?
If a switch statement does not have a break keyword in one of the cases, the program will “fall through” and continue executing the subsequent case statements, even if their condition doesn’t match. This behavior is often used intentionally in some cases but can lead to unintended results if not handled properly.
Example without break: program in Java
public class SwitchFallThroughExample {
public static void main(String[] args) {
int day = 3;
switch (day) {
case 1:
System.out.println(“Monday”);
break;
case 2:
System.out.println(“Tuesday”);
break;
case 3:
System.out.println(“Wednesday”);
case 4:
System.out.println(“Thursday”);
break;
case 5:
System.out.println(“Friday”);
break;
default:
System.out.println(“Invalid day”);
}
}
}
Output:
Wednesday
Thursday
In this example, since case 3 does not have a break, the program falls through to case 4 and prints both “Wednesday” and “Thursday.”
21. What is a method in Java? How is it different from a function?
A method in Java is a block of code that performs a specific task and is associated with an object or class. It has a name, a return type, and can accept parameters. In Java, methods are defined inside a class, and they define behaviors or actions that an object or class can perform.
A function, in general programming, is a block of code designed to perform a particular task, but in Java, the term “function” is used less frequently compared to “method.”
Difference:
- A method is part of an object or class in Java, whereas a function is not necessarily associated with an object.
- Java does not allow standalone functions outside of classes, so all methods are part of a class or object.
22. Explain the difference between static and non-static methods in Java.
- Static Method:
- Belongs to the class rather than an instance of the class.
- Can be called without creating an instance of the class.
- Can only access static members of the class (variables or other methods).
- Non-Static Method (Instance Method):
- Belongs to an instance of the class.
- Must be called by creating an instance of the class.
- Can access both static and non-static members of the class.
Example: program in Java
public class Example {
static int staticVar = 10;
int instanceVar = 20;
// Static method
public static void staticMethod() {
System.out.println(“Static Method: ” + staticVar);
}
// Non-static method
public void instanceMethod() {
System.out.println(“Instance Method: ” + instanceVar);
}
public static void main(String[] args) {
// Calling static method without an instance
staticMethod();
// Calling non-static method requires an instance
Example obj = new Example();
obj.instanceMethod();
}
}
23. Write a program to calculate the sum of two numbers using a method.
Program in Java
import java.util.Scanner;
public class SumExample {
// Method to calculate the sum
public static int sum(int a, int b) {
return a + b;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter first number: “);
int num1 = scanner.nextInt();
System.out.print(“Enter second number: “);
int num2 = scanner.nextInt();
// Calling the method to get the sum
int result = sum(num1, num2);
System.out.println(“Sum: ” + result);
}
}
24. What is method overloading? Provide an example.
Method Overloading occurs when two or more methods in the same class have the same name but differ in parameters (either by number or type of arguments).
Example: program in Java
public class OverloadExample {
// Overloaded method with two integer parameters
public int add(int a, int b) {
return a + b;
}
// Overloaded method with three integer parameters
public int add(int a, int b, int c) {
return a + b + c;
}
public static void main(String[] args) {
OverloadExample obj = new OverloadExample();
System.out.println(“Sum of two numbers: ” + obj.add(10, 20));
System.out.println(“Sum of three numbers: ” + obj.add(10, 20, 30));
}
}
25. How do you pass arguments to a method by value in Java?
In Java, method arguments are passed by value. This means that when you pass a variable to a method, the method works on a copy of the original variable and cannot modify the original variable.
Example: program in Java
public class PassByValueExample {
public static void changeValue(int num) {
num = 10; // This change will not affect the original value
}
public static void main(String[] args) {
int number = 5;
changeValue(number); // Passing by value
System.out.println(“Number after method call: ” + number); // It will still be 5
}
}
26. Write (program in Java) a method in Java to check whether a given number is prime.
public class PrimeCheck {
public static boolean isPrime(int num) {
if (num <= 1) {
return false;
}
for (int i = 2; i < num; i++) {
if (num % i == 0) {
return false;
}
}
return true;
}
public static void main(String[] args) {
int number = 17;
if (isPrime(number)) {
System.out.println(number + ” is prime.”);
} else {
System.out.println(number + ” is not prime.”);
}
}
}
Read also: C Language Interview Questions And Answers: Best Interview QA in 2024
27. What is the return type of a method? Explain with examples.
The return type of a method specifies the type of value the method will return after it is executed. It can be any valid data type (e.g., int, String, double, etc.), or void if the method does not return anything.
Example: program in Java
public class ReturnTypeExample {
// Method with return type ‘int’
public static int getSquare(int num) {
return num * num;
}
// Method with return type ‘void’ (doesn’t return anything)
public static void printMessage() {
System.out.println(“Hello from Java!”);
}
public static void main(String[] args) {
int square = getSquare(5);
System.out.println(“Square of 5: ” + square);
printMessage(); // Calling void method
}
}
28. Write a program in Java that demonstrates a method with no arguments and no return value.
public class NoArgumentsNoReturn {
public static void greet() {
System.out.println(“Hello! This is a method with no arguments and no return value.”);
}
public static void main(String[] args) {
greet(); // Calling the method with no arguments and no return value
}
}
29. How can methods help in modular programming? Provide an example.
Methods help in modular programming by allowing you to break down a complex program into smaller, manageable chunks of code. This makes the program easier to understand, maintain, and reuse.
Example: program in Java
public class ModularExample {
public static void printGreeting() {
System.out.println(“Hello, welcome to modular programming!”);
}
public static void printFarewell() {
System.out.println(“Goodbye, see you again!”);
}
public static void main(String[] args) {
printGreeting();
printFarewell();
}
}
In this example, the program is divided into smaller methods (printGreeting and printFarewell), each performing a specific task.
30. Write a program to calculate the greatest common divisor (GCD) of two numbers using a method.
public class GCDExample {
public static int gcd(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
public static void main(String[] args) {
int num1 = 56, num2 = 98;
int result = gcd(num1, num2);
System.out.println(“GCD of ” + num1 + ” and ” + num2 + ” is: ” + result);
}
}
31. Explain the difference between call-by-value and call-by-reference with respect to Java methods.
- Call-by-value: In Java, all method arguments are passed by value, meaning the actual value is passed to the method. Changes to the parameter inside the method do not affect the original value.
- Call-by-reference: Java does not support call-by-reference directly, but it simulates this with objects. When passing an object to a method, the reference to the object is passed, allowing the method to modify the object itself.
32. Write(program in Java) a method in Java to reverse a string.
public class StringReverse {
public static String reverseString(String str) {
String reversed = “”;
for (int i = str.length() – 1; i >= 0; i–) {
reversed += str.charAt(i);
}
return reversed;
}
public static void main(String[] args) {
String original = “Java Programming”;
String reversed = reverseString(original);
System.out.println(“Reversed String: ” + reversed);
}
}
33. What is the purpose of the main method in Java?
The main method in Java serves as the entry point for program execution. It is the method that the Java Virtual Machine (JVM) calls when running the program. Without the main method, a Java program cannot run.
Program in Java
public class MainMethodExample {
public static void main(String[] args) {
System.out.println(“This is the entry point of the Java program.”);
}
}
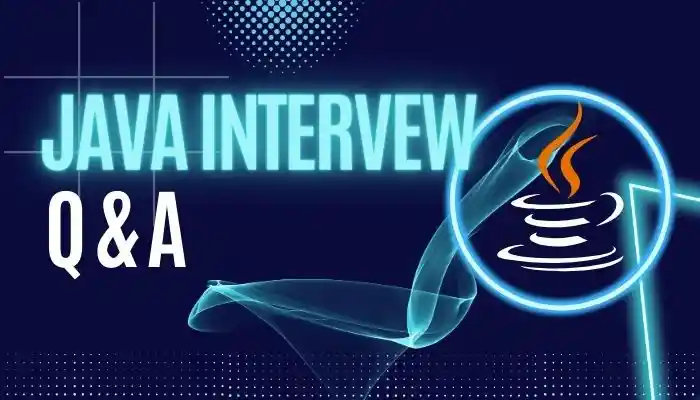
34. Explain the use of recursion in Java methods. Provide an example.
Recursion occurs when a method calls itself. It is typically used for problems that can be broken down into smaller subproblems of the same type.
Example (Factorial): program in Java
public class RecursionExample {
public static int factorial(int n) {
if (n == 0) {
return 1; // Base case
} else {
return n * factorial(n – 1); // Recursive call
}
}
public static void main(String[] args) {
int result = factorial(5);
System.out.println(“Factorial of 5 is: ” + result);
}
}
Read also: Essential Guide to Prompt Engineering: Tools, Techniques, Roadmap, Best Courses in 2024
35. Write (program in Java) a method to find the maximum value in an array of integers.
public class MaxInArray {
public static int findMax(int[] arr) {
int max = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
public static void main(String[] args) {
int[] numbers = {12, 56, 3, 89, 24};
int maxVal = findMax(numbers);
System.out.println(“Maximum value: ” + maxVal);
}
}
36. What is an array in Java, and how is it different from a variable?
An array in Java is a data structure that stores multiple values of the same data type in a contiguous memory location. It is a reference type and can hold multiple elements, such as integers, strings, or objects. A variable, on the other hand, can hold only a single value of a particular data type.
Difference:
- An array can hold multiple values, while a variable holds only one value.
- Arrays are objects in Java, while variables are basic data types or references.
37. How do you declare, initialize, and access elements in an array? Provide an example.
- Declaration: Specifies the type of elements and the name of the array.
- Initialization: Defines the actual array with values.
- Accessing elements: Use the index to access or modify elements.
Example: program in Java
public class ArrayExample {
public static void main(String[] args) {
// Declare and initialize an array of integers
int[] numbers = {10, 20, 30, 40, 50};
// Access elements using index
System.out.println(“First element: ” + numbers[0]); // 10
System.out.println(“Third element: ” + numbers[2]); // 30
}
}

38. Write a program in Java to find the largest and smallest numbers in an array.
public class LargestSmallestExample {
public static void main(String[] args) {
int[] numbers = {10, 2, 35, 40, 5, 100};
int largest = numbers[0];
int smallest = numbers[0];
for (int num : numbers) {
if (num > largest) {
largest = num;
}
if (num < smallest) {
smallest = num;
}
}
System.out.println(“Largest number: ” + largest);
System.out.println(“Smallest number: ” + smallest);
}
}
39. Explain the difference between single-dimensional and multi-dimensional arrays in Java.
- Single-dimensional array: A simple list of elements, like a row of data.
- Example: int[] arr = {1, 2, 3, 4, 5};
- Multi-dimensional array: An array that contains arrays, which can represent a matrix or grid of data. It can have two or more dimensions.
- Example: int[][] matrix = {{1, 2}, {3, 4}, {5, 6}}; (2D array)
40. Write a program in Java to add two matrices using 2D arrays.
public class MatrixAddition {
public static void main(String[] args) {
int[][] matrix1 = {
{1, 2, 3},
{4, 5, 6}
};
int[][] matrix2 = {
{7, 8, 9},
{10, 11, 12}
};
int[][] result = new int[2][3]; // Resultant matrix
// Add matrices
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
result[i][j] = matrix1[i][j] + matrix2[i][j];
}
}
// Display result
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
System.out.print(result[i][j] + ” “);
}
System.out.println();
}
}
}
41. What is the default value of elements in an array of integers, floats, and strings?
- Integer Array: Default value is 0.
- Float Array: Default value is 0.0.
- String Array: Default value is null.
Example: program in Java
public class DefaultValues {
public static void main(String[] args) {
int[] intArray = new int[3];
float[] floatArray = new float[3];
String[] stringArray = new String[3];
System.out.println(“Default int value: ” + intArray[0]);
System.out.println(“Default float value: ” + floatArray[0]);
System.out.println(“Default string value: ” + stringArray[0]);
}
}
42. Write a program in Java to calculate the average of numbers in an array.
public class AverageExample {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
int sum = 0;
for (int num : numbers) {
sum += num;
}
double average = sum / (double) numbers.length;
System.out.println(“Average: ” + average);
}
}
43. How do you copy elements from one array to another in Java? Write a program in Java.
import java.util.Arrays;
public class ArrayCopy {
public static void main(String[] args) {
int[] original = {1, 2, 3, 4, 5};
int[] copy = new int[original.length];
// Using Arrays.copyOf method to copy elements
copy = Arrays.copyOf(original, original.length);
// Displaying copied array
System.out.println(“Copied array: ” + Arrays.toString(copy));
}
}
44. Explain the purpose of the Arrays class in Java. Provide examples of its methods (sort, binarySearch, etc.).
The Arrays class in Java provides various utility methods for working with arrays, including sorting, searching, filling, and converting arrays.
- sort(): Sorts the array.
- binarySearch(): Performs a binary search to find the index of an element.
- toString(): Converts the array to a string representation.
Example: program in Java
import java.util.Arrays;
public class ArraysExample {
public static void main(String[] args) {
int[] arr = {5, 3, 8, 1, 2};
// Sorting the array
Arrays.sort(arr);
System.out.println(“Sorted array: ” + Arrays.toString(arr));
// Binary search for an element
int index = Arrays.binarySearch(arr, 3);
System.out.println(“Index of 3: ” + index);
}
}
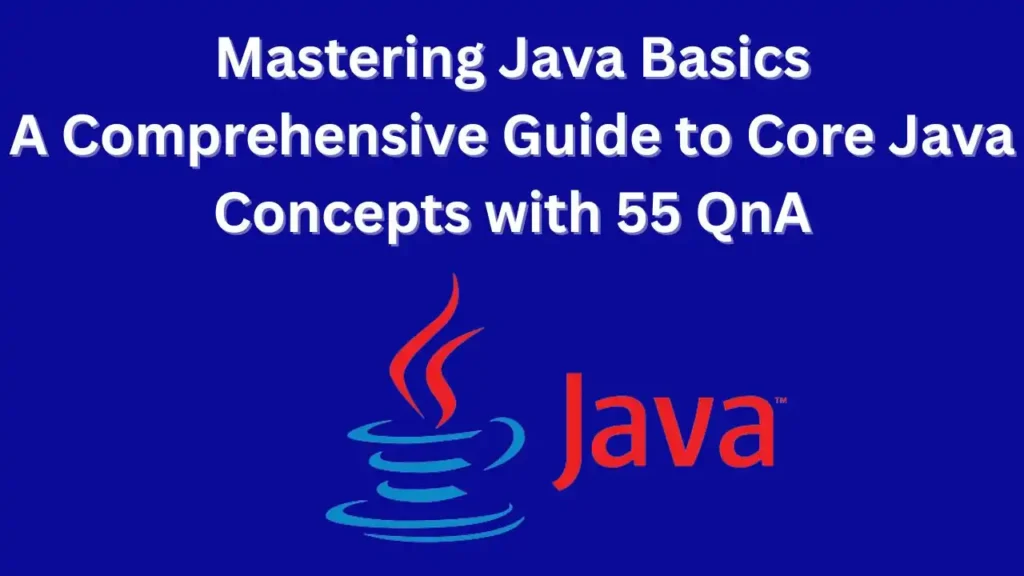
45. Write a program in Java to check if an array contains a specific value.
import java.util.Arrays;
public class ArrayContainsExample {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
// Check if array contains 30
boolean contains = Arrays.stream(numbers).anyMatch(num -> num == 30);
System.out.println(“Array contains 30: ” + contains);
}
}
46. How can you reverse an array in Java? Write a program in Java.
public class ArrayReverse {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
int start = 0;
int end = numbers.length – 1;
while (start < end) {
// Swap the elements
int temp = numbers[start];
numbers[start] = numbers[end];
numbers[end] = temp;
start++;
end–;
}
// Print reversed array
for (int num : numbers) {
System.out.print(num + ” “);
}
}
}
47. Write a program in Java to remove duplicate elements from an array.
import java.util.Arrays;
import java.util.HashSet;
public class RemoveDuplicates {
public static void main(String[] args) {
int[] numbers = {10, 20, 20, 30, 40, 40, 50};
HashSet<Integer> set = new HashSet<>();
// Add elements to the set to remove duplicates
for (int num : numbers) {
set.add(num);
}
// Convert set back to array
Integer[] uniqueNumbers = set.toArray(new Integer[0]);
// Print unique elements
System.out.println(“Array without duplicates: ” + Arrays.toString(uniqueNumbers));
}
}
48 How do you iterate through an array using an enhanced for loop? Provide an example program in Java.
public class EnhancedForLoopExample {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
// Enhanced for loop to iterate through array
for (int num : numbers) {
System.out.print(num + ” “);
}
}
}
49. Write a program in Java to find the sum of the diagonal elements of a 2D array.
public class DiagonalSum {
public static void main(String[] args) {
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int sum = 0;
for (int i = 0; i < matrix.length; i++) {
sum += matrix[i][i]; // Sum of diagonal elements
}
System.out.println(“Sum of diagonal elements: ” + sum);
}
}
50. How is a String array different from an int array in Java? Provide examples.
- A String array stores strings, which are objects in Java.
- An int array stores primitive integer values.
Example: program in Java
public class ArrayTypes {
public static void main(String[] args) {
String[] strArray = {“apple”, “banana”, “cherry”};
int[] intArray = {1, 2, 3, 4, 5};
System.out.println(“String array: ” + Arrays.toString(strArray));
System.out.println(“Int array: ” + Arrays.toString(intArray));
}
}
51. Write a program in Java to merge two arrays into a single array.
import java.util.Arrays;
public class MergeArrays {
public static void main(String[] args) {
int[] array1 = {1, 2, 3};
int[] array2 = {4, 5, 6};
int[] mergedArray = new int[array1.length + array2.length];
// Copy elements of array1
System.arraycopy(array1, 0, mergedArray, 0, array1.length);
// Copy elements of array2
System.arraycopy(array2, 0, mergedArray, array1.length, array2.length);
// Display merged array
System.out.println(“Merged array: ” + Arrays.toString(mergedArray));
}
}
52. What are jagged arrays in Java? Explain with an example.
A jagged array is an array of arrays where each “inner” array can have different lengths.
Example:
public class JaggedArrayExample {
public static void main(String[] args) {
int[][] jaggedArray = {
{1, 2, 3},
{4, 5},
{6, 7, 8, 9}
};
// Display jagged array elements
for (int i = 0; i < jaggedArray.length; i++) {
for (int j = 0; j < jaggedArray[i].length; j++) {
System.out.print(jaggedArray[i][j] + ” “);
}
System.out.println();
}
}
}
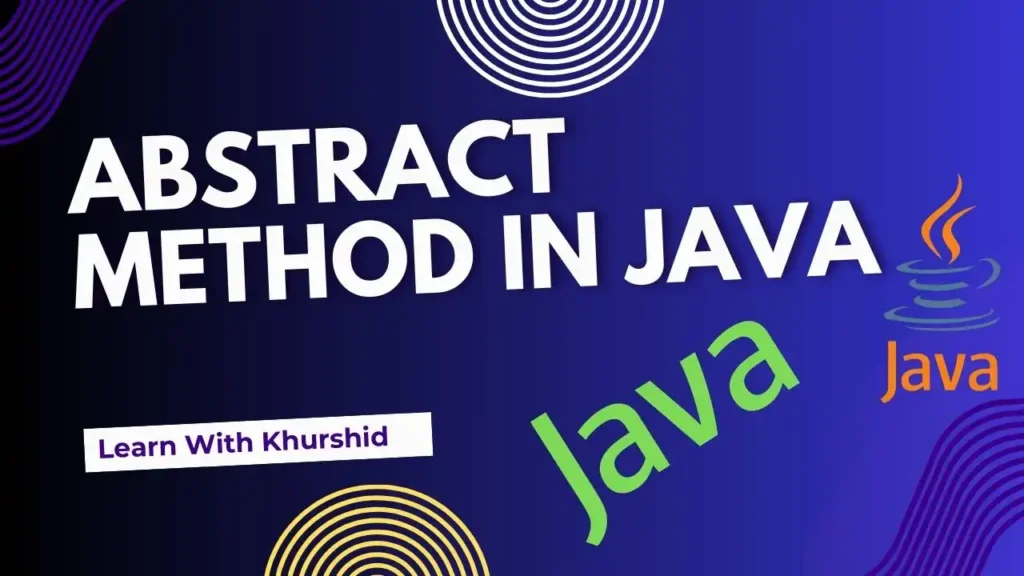
53. Write a program in Java to find the frequency of each element in an array.
import java.util.HashMap;
public class FrequencyCount {
public static void main(String[] args) {
int[] numbers = {10, 20, 20, 30, 30, 30, 40};
HashMap<Integer, Integer> frequencyMap = new HashMap<>();
for (int num : numbers) {
frequencyMap.put(num, frequencyMap.getOrDefault(num, 0) + 1);
}
// Print frequency of each element
System.out.println(“Frequency of elements: ” + frequencyMap);
}
}
54. How do you sort an array in descending order? Write a program in Java.
import java.util.Arrays;
import java.util.Collections;
public class DescendingSort {
public static void main(String[] args) {
Integer[] numbers = {10, 20, 30, 40, 50};
// Sort array in descending order
Arrays.sort(numbers, Collections.reverseOrder());
// Display sorted array
System.out.println(“Sorted array (descending): ” + Arrays.toString(numbers));
}
}
55. Write a program in Java to rotate an array to the left or right by a given number of positions.
public class ArrayRotation {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
int positions = 2; // Rotate to the right by 2 positions
// Rotate array
for (int i = 0; i < positions; i++) {
int temp = numbers[numbers.length – 1];
for (int j = numbers.length – 1; j > 0; j–) {
numbers[j] = numbers[j – 1];
}
numbers[0] = temp;
}
// Display rotated array
System.out.println(“Array after rotation: ” + Arrays.toString(numbers));
}
}